Checkbox
Checkbox allows to select one or more items from a group, or switch between two mutually exclusive options (checked or unchecked, on or off).
Examples
Basic checkboxes
- Python
import flet as ft
def main(page):
def button_clicked(e):
t.value = (
f"Checkboxes values are: {c1.value}, {c2.value}, {c3.value}, {c4.value}, {c5.value}."
)
page.update()
t = ft.Text()
c1 = ft.Checkbox(label="Unchecked by default checkbox", value=False)
c2 = ft.Checkbox(label="Undefined by default tristate checkbox", tristate=True)
c3 = ft.Checkbox(label="Checked by default checkbox", value=True)
c4 = ft.Checkbox(label="Disabled checkbox", disabled=True)
c5 = ft.Checkbox(
label="Checkbox with rendered label_position='left'", label_position=ft.LabelPosition.LEFT
)
b = ft.ElevatedButton(text="Submit", on_click=button_clicked)
page.add(c1, c2, c3, c4, c5, b, t)
ft.app(main)
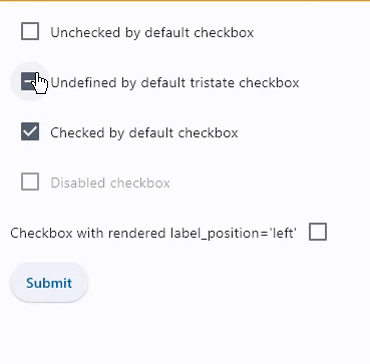
Checkbox with on_change
event
- Python
import flet as ft
def main(page):
def checkbox_changed(e):
t.value = f"Checkbox value changed to {c.value}"
t.update()
c = ft.Checkbox(label="Checkbox with 'change' event", on_change=checkbox_changed)
t = ft.Text()
page.add(c, t)
ft.app(main)
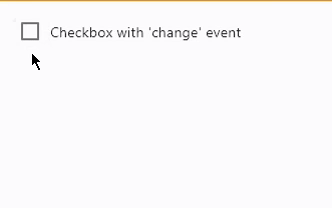
Properties
active_color
The color to use when this checkbox is checked.
adaptive
If the value is True
, an adaptive Checkbox is created based on whether the target platform is iOS/macOS.
On iOS and macOS, a CupertinoCheckbox
is created, which has matching functionality and presentation as Checkbox
, and the graphics as expected on iOS. On other platforms, a Material Checkbox is created.
See the example of usage here.
Defaults to False
.
autofocus
True if the control will be selected as the initial focus. If there is more than one control on a page with autofocus set, then the first one added to the page will get focus.
border
The color and width of the checkbox's border to be rendered when the checkbox's value
is False
.
check_color
The color to use for the check icon when this checkbox is checked.