Standalone Flet web apps with Pyodide
We've just released Flet 0.4.0 with a super exciting new feature - packaging Flet apps into a standalone static website that can be run entirely in the browser! The app can be published to any free hosting for static websites such as GitHub Pages or Cloudflare Pages. Thanks to Pyodide - a Python port to WebAssembly!
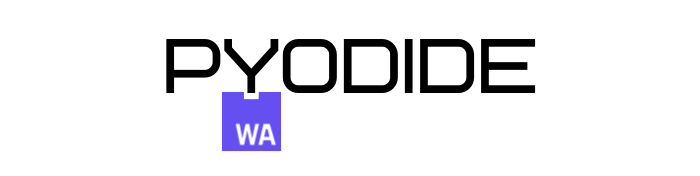
You can quickly build awesome single-page applications (SPA) entirely in Python and host them everywhere! No HTML, CSS or JavaScript required!
Quick Flet with Pyodide demo
Install the latest Flet package:
pip install flet --upgrade
Create a simple counter.py
app:
import flet as ft
def main(page: ft.Page):
page.title = "Flet counter example"
page.vertical_alignment = ft.MainAxisAlignment.CENTER
txt_number = ft.TextField(value="0", text_align=ft.TextAlign.RIGHT, width=100)
def minus_click(e):
txt_number.value = str(int(txt_number.value) - 1)
page.update()
def plus_click(e):
txt_number.value = str(int(txt_number.value) + 1)
page.update()
page.add(
ft.Row(
[
ft.IconButton(ft.icons.REMOVE, on_click=minus_click),
txt_number,
ft.IconButton(ft.icons.ADD, on_click=plus_click),
],
alignment=ft.MainAxisAlignment.CENTER,
)
)
ft.app(main)
Run a brand-new flet publish
command to publish Flet app as a static website:
flet publish counter.py
The website will be published to dist
directory next to counter.py
.
Give website a try using built-in Python web server:
python -m http.server --directory dist
Open http://localhost:8000
in your browser to check the published app.
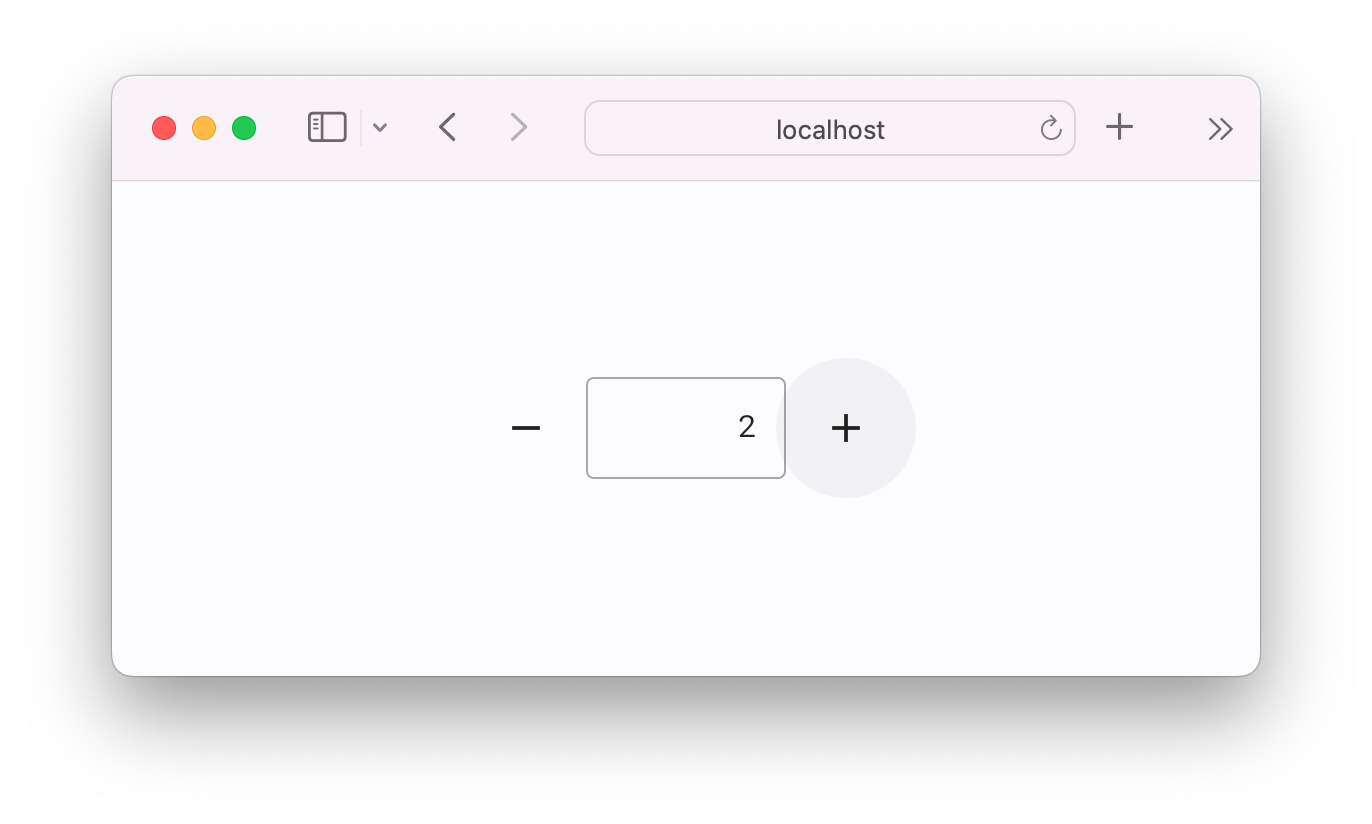
Here are a few live Flet apps hosted at Cloudflare Pages:
Check the guide for more information about publishing Flet apps as standalone websites.
Built-in Fletd server in Python
Flet 0.4.0 also implements a new Flet desktop architecture.
It replaces Fletd server written in Go with a light-weight shim written in Python with a number of pros:
- Only 2 system processes are needed to run Flet app: Python interpreter and Flutter client.
- Less communication overhead (minus two network hops between Python and Fletd) and lower latency (shim uses TCP on Windows and Unix domain sockets on macOS/Linux).
- Shim binds to
127.0.0.1
on Windows by default which is more secure. - The size of a standalone app bundle produced by
flet pack
reduced by ~8 MB.
The implementation was also required to support Pyodide (we can't run Go web server in the browser, right?) and paves the way to iOS and Android support.
Other changes
- All controls loading resources from web URLs (
Image.src
,Audio.src
,Page.fonts
,Container.image_src
) are now able to load them from local files too, by providing a full path in the file system, and fromassets
directory by providing relative path. For desktop apps a path insrc
property could be one of the following:- A path relative to
assets
directory, with or without starting slash, for example:/image.png
orimage.png
. The name of artifact dir should not be included. - An absolute path within a computer file system, e.g.
C:\projects\app\assets\image.png
or/Users/john/images/picture.png
. - A full URL, e.g.
https://mysite.com/images/pic.png
. - Add
page.on_error = lambda e: print("Page error:", e.data)
to see failing images.
- A path relative to
flet
Python package has separated into two packages:flet-core
andflet
.- PDM replaced with Poetry.
beartype
removed everywhere.
💥 Breaking changes
- Default routing scheme changed from "hash" to "path" (no
/#/
at the end of app URL). Useft.app(main, route_url_strategy="hash")
to get original behavior. - OAuth authentication is not supported anymore in standalone desktop Flet apps.
Async support
Flet apps can now be written as async apps and use asyncio
with other Python async libraries. Calling coroutines is naturally supported in Flet, so you don't need to wrap them to run synchronously.
To start with an async Flet app you should make main()
method async
:
import flet as ft
async def main(page: ft.Page):
await page.add_async(ft.Text("Hello, async world!"))
ft.app(main)
Read the guide for more information about writing async Flet apps.
Conclusion
Flet 0.4.0 brings the following exciting features:
- Standalone web apps with Pyodide running in the browser and hosted on a cheap hosting.
- Faster and more secure architecture with a built-in Fletd server.
- Async apps support.
Upgrade Flet module to the latest version (pip install flet --upgrade
), give flet publish
command a try and let us know what you think!
Hey, by the way, Flet project has reached ⭐️ 4.2K stars ⭐️ (+1K in just one month) - keep going!