ShaderMask
A control that applies a mask generated by a shader to its child.
For example, ShaderMask
can be used to gradually fade out the edge of a child by using a LinearGradient
mask.
Examples
Adding a pink glow around image edges
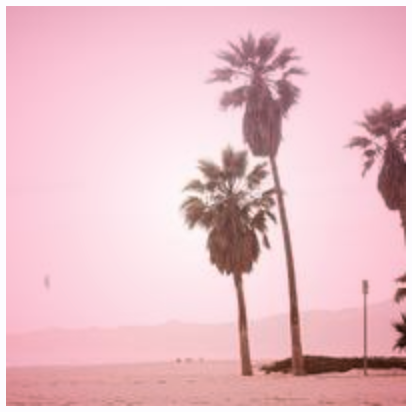
- Python
import flet as ft
def main(page: ft.Page):
page.add(
ft.Row(
[
ft.ShaderMask(
ft.Image(
src="https://picsum.photos/200/200?1",
width=200,
height=200,
fit=ft.ImageFit.FILL,
),
blend_mode=ft.BlendMode.MULTIPLY,
shader=ft.RadialGradient(
center=ft.alignment.center,
radius=2.0,
colors=[ft.Colors.WHITE, ft.Colors.PINK],
tile_mode=ft.GradientTileMode.CLAMP,
),
)
]
)
)
ft.app(main)
Gradually fade out image to the bottom edge
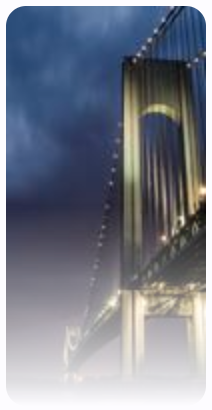
- Python
import flet as ft
def main(page: ft.Page):
page.add(
ft.Row(
[
ft.ShaderMask(
ft.Image(src="https://picsum.photos/100/200?2"),
blend_mode=ft.BlendMode.DST_IN,
shader=ft.LinearGradient(
begin=ft.alignment.top_center,
end=ft.alignment.bottom_center,
colors=[ft.Colors.BLACK, ft.Colors.TRANSPARENT],
stops=[0.5, 1.0],
),
border_radius=10,
),
]
)
)
ft.app(main)
Properties
blend_mode
The blend mode to use when applying the shader to the content
.
Value is of type BlendMode
and defaults to BlendMode.MODULATE
.
border_radius
The radius of the mask.
Value is of type BorderRadius
.
content
A child Control
to apply a shader to.
shader
Use gradient as a shader.
Value is of type Gradient
.