Ads
Displays ads in your app. Only available for mobile (Android and iOS) platforms.
Packaging
To build your Flet app that uses ads control add flet-ads
to dependencies
key of the [project]
section of your pyproject.toml
file, for
example:
[project]
...
dependencies = [
"flet==0.27.6",
"flet-ads==0.1.0",
]
Examples
python/controls/information-displays/ads/ads-basic-example.py
loading...
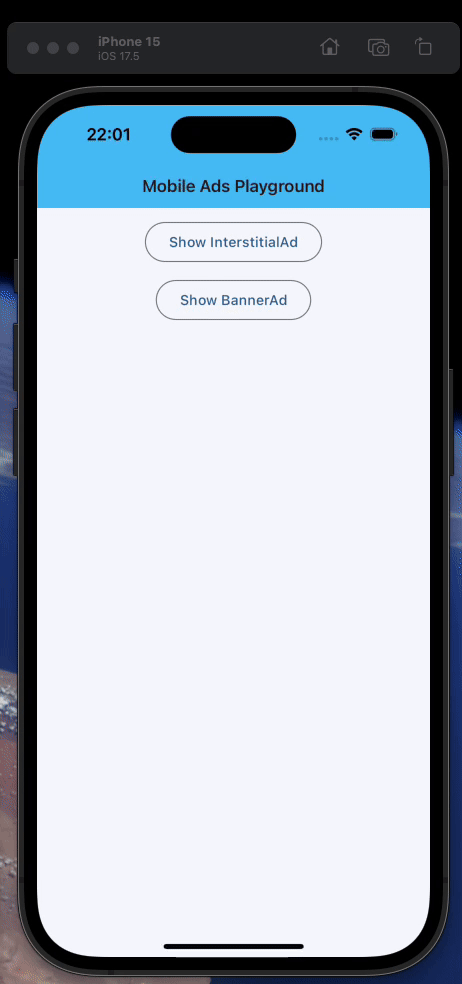
Types
The following types are available:
Test Values
AdMob provides app and ad unit IDs for testing purposes.
- AdMob app ID:
"ca-app-pub-3940256099942544~3347511713"
BannerAd.unit_id
on Android:"ca-app-pub-3940256099942544/9214589741"
BannerAd.unit_id
on iOS:"ca-app-pub-3940256099942544/2435281174"
InterstitialAd.unit_id
on Android:"ca-app-pub-3940256099942544/1033173712"
InterstitialAd.unit_id
on iOS:"ca-app-pub-3940256099942544/4411468910"
Remember to replace these values with your own when you're ready to package your app.
Packaging
The following are to be done when packaging an app that makes use of one of the above ad controls.
Include Ads package
Add flet-ads
to dependencies
key of the [project]
section of your pyproject.toml
configuration file, for example:
[project]
...
dependencies = [
...
"flet-ads==0.1.0",
...
]
Specify AdMob app ID
Specify your AdMob app ID, without which your application might crash on launch.
You can specify the app ID in two ways:
- In your
pyproject.toml
file:
# for Android
[tool.flet.android.meta_data]
"com.google.android.gms.ads.APPLICATION_ID" = "ca-app-pub-xxxxxxxxxxxxxxxx~yyyyyyyyyy"
# for iOS
[tool.flet.ios.info]
GADApplicationIdentifier = "ca-app-pub-xxxxxxxxxxxxxxxx~yyyyyyyyyy"
- In your build command from the terminal:
# for Android
flet build apk ... --android-meta-data com.google.android.gms.ads.APPLICATION_ID=ca-app-pub-xxxxxxxxxxxxxxxx~yyyyyyyyyy
# for iOS
flet build ipa ... --info-plist GADApplicationIdentifier=ca-app-pub-xxxxxxxxxxxxxxxx~yyyyyyyyyy